From my experience, many Coders are wizards at writing code, but they often play it cool when it comes to sharing their trade secrets. Sometimes, it's because they only don't know the secret behind the tools they use. Today, let's explore one of the most powerful tools being used daily – The Java Virtual Machine (JVM).
How does JVM actually Work ?
The Java Virtual Machine or JVM is your code's best friend, turning Java programs into Machine code that can run on any Operating System or Device which has Java implementation.
(i) Writing the Code: The main code for execution is typed in any software such as BlueJ or VSC with ".java" extension.
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
(ii) Compile the Code: The Java Compiler or Javac converts the .java files into Bytecode.
(iii) Execution of Code: The JVM reads the ".class" files and converts the Bytecode into Machine Code for the required system hardware.
(iv) Output:
Hello, World!
Key Features of JVM
(i) Huge Ecosystem: The JVM doesn't only support Java but besides that it also supports other languages such as Scala and Kotlin, which are still used in the modern world.
(ii) Platform Independent: You can write a program once and run it anywhere on any software, hardware without worrying about the operating system. This is what the JVM offers to its users - Freedom !
(iii) The Garbage Collector: The garbage collector identifies objects that cannot be used by active or static media and removes them to free up memory. This feature prevents memory leaks and lowers the chance of running out of memory. This feature falls under JVM Memory Management.
(iv) Memory Management: It involves the allocation and deallocation of memory to various parts of a program. It involves handling the stack, heap, and method area, ensuring that each part of the JVM's memory is used effectively.
(v) Security: Think of the JVM as your Application's personal Bodyguard, equipped with high-tech defenses to shield against sneaky Cyber Intruders and keep your system safe from any digital threats.
(vi) Performance Optimization: The JVM includes a Just-In-Time (JIT) compiler that converts bytecode to machine code at runtime, which improves the performance of Java applications. It is explained down in much detail !
Memory Management in JVM
- Heap: This is where all the objects and their corresponding data are stored like a real-life heap.
- Stack: It stores local variables, method parameters, and the call stack (stores information about Methods or Functions).
- Method Area: This stores class data, including information about the class structure, static variables and the code for Methods or Functions.
- Garbage Collector: The garbage collector is an automatic memory management system in the JVM that identifies memory occupied by objects no longer in use, preventing memory leaks and optimizing proper resource usage.
public class MemoryManagement
{
public static void main(String[] args)
{
String name = new String("Ramesh Agrawal");
Integer age = new Integer(30);
System.out.println("Name: " + name);
System.out.println("Age: " + age);
//JVM handles garbage collection
//No need to explicitly deallocate memory manually
}
}
Heap:
- The String object "Ramesh Agrawal".
- The Integer object 30 is also created in the heap.
Stack:
- The reference variable name, which points to the String object "Ramesh Agrawal" in the heap.
- The reference variable age, which points to the Integer object 30 in the heap.
- The println method create their own Stack as well.
Method Area:
- The main MemoryManagement class, includes information about its methods (Eg. main) is stored in the method area.
- The String and Integer classes' information are stored in the method area.
- Static variables and Constants would also be stored here, in Method Area.
JVM Garbage Collector:
- After the main method completes, the stack frames for main and its local variables (name and age) are removed. At this point, name and age are no longer active. The JVM detects that these objects (String and Integer) and thus are no longer referenced for next method.
JIT: For Lightning Fast Performance
Let's talk about JIT (Just-In-Time) compilation – the secret sauce that makes your Java programs go boom ! Imagine your code getting an on-the-fly upgrade right before it runs. That's JIT!
JIT keeps your apps running smooth and swift by optimizing critical loops and functions. It improves performance by converting Bytecode into native Machine Code at runtime, allowing code paths to be optimized for faster execution. It identifies certain Section of code such as Variables, optimizing them more for better Speed. This process balances the Code with better performance outputs.
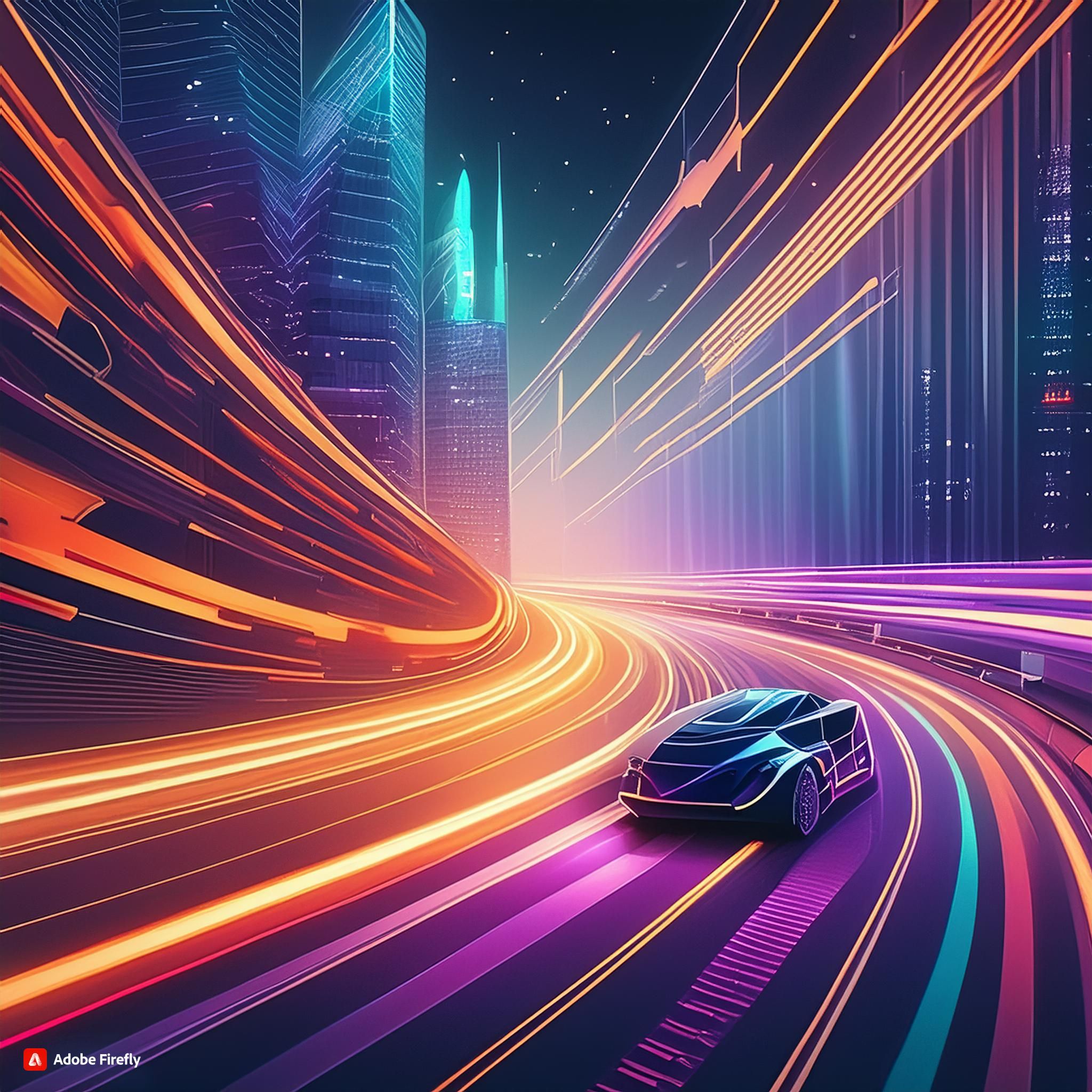
Conclusion for Today
Java Virtual Machine (JVM) is more than just a tool for running Java programs. It provides a secure and flexible environment that allows developers to create applications that can run on any device.
Learning how the JVM works is like unlocking Java's secret powers. Whether you're a newbie or a pro, grasping the JVM is the key to building an awesome software that rocks for the future !