Have you ever wondered why arrays in programming languages like Java, C++, and many others start counting from 0 instead of 1? I mean, it goes against the conventional way we count things, right? Well, let me share some insights I've gathered along my programming journey.
Back in the 9th grade, when I first started programming in Java, I learned about arrays. Like many of you, I was puzzled by why the indexing started at 0. It seemed counterintuitive at first, but as I dived deeper into the world of programming, I stumbled upon the rationale behind this design choice(Thanks to the C++ course and Google!).
The main reason behind starting arrays at 0 is rooted in language design and, more importantly, memory efficiency. Let's break it down a bit.
When we create an array in a programming language, behind the scenes, the computer allocates a contiguous block of memory to hold all the elements of that array. Each element occupies a certain amount of space in memory, and the index is essentially an offset from the beginning of the array.
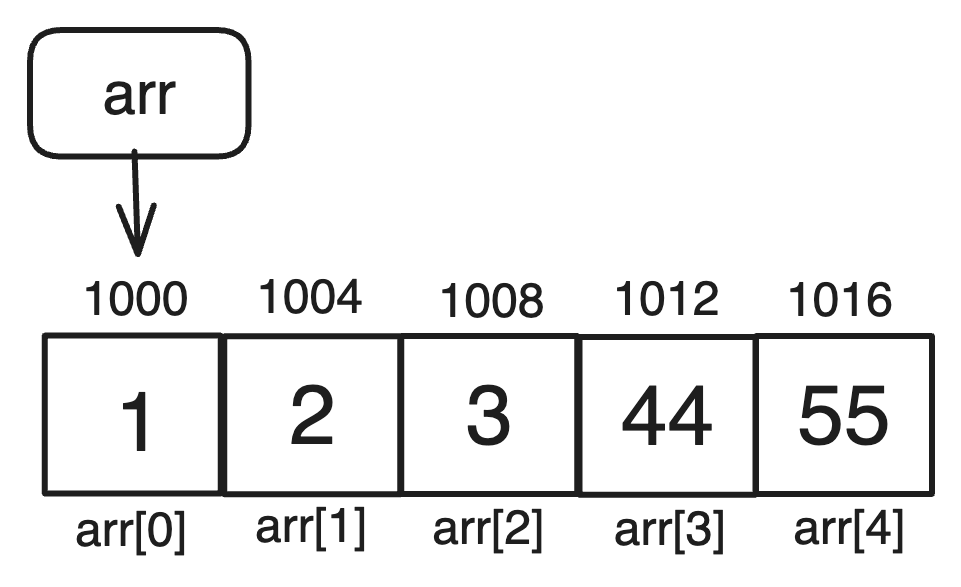
Now, here's where things get interesting. In languages like C and C++, arrays are often used in conjunction with pointers. In fact, arrays and pointers are intimately related in these languages. When you access an element of an array, say arr[i], the compiler translates it into something like *(arr + i), where arr is the base address of the array.
By starting the indexing at 0, the first element of the array is at an offset of 0 from the base address. This simplifies the pointer arithmetic and aligns with the underlying memory representation. It's like saying, "Hey computer, give me the element that's 0 offsets away from the start of this array."
But why not start at 1, you might ask? Well, doing so would require an extra operation in the pointer arithmetic. You'd have to subtract 1 from the index to get the correct offset, adding unnecessary overhead and complexity. By starting at 0, we keep things simple and efficient.